Zdog — 3D javascript engine

Just found that very interesting 3D javascript engine for canvas and SVG.
You can design and render 3D models on the web via using Zdog.
Zdog is small. 2,100 lines of code for the entire library. 28KB minified.
Zdog is round. All circular shapes are rendered as proper circles with rounded edges. No polygonal jaggies.
Zdog is friendly. Modeling is done with a straight-forward declarative API.
Without the further adoo, Let’s get started.
CDN (link directly to Zdog JS on unpkg)
<script src="https://unpkg.com/zdog@1/dist/zdog.dist.min.js"></script>
or you can use Package manager
- Install with npm:
npm install zdog
- Install with Bower:
bower install zdog --save
Getting Started
Add pkg in index.html
<script src="https://unpkg.com/zdog@1/dist/zdog.dist.min.js"></script>
Copy and paste the hello world demo
let isSpinning = true;
let illo = new Zdog.Illustration({
element: '.zdog-canvas',
dragRotate: true,
// stop spinning when drag starts
onDragStart: function() {
isSpinning = false;
},
});
// circle
new Zdog.Ellipse({
addTo: illo,
diameter: 80,
translate: { z: 40 },
stroke: 20,
color: '#636',
});
// square
new Zdog.Rect({
addTo: illo,
width: 80,
height: 80,
translate: { z: -40 },
stroke: 12,
color: '#E62',
fill: true,
});
function animate() {
illo.rotate.y += isSpinning ? 0.03 : 0;
illo.updateRenderGraph();
requestAnimationFrame( animate );
}
animate();
Modeling
Child Shapes
- Shapes can be positioned with translate css property.
- Positions are relative -> applying to child shapes

let zCircle = new Zdog.Ellipse({
addTo: illo,
translate: { z: 40 }, // z +40 from illo
// ...
});
let xRect = new Zdog.Rect({
addTo: illo,
translate: { x: 40 }, // x +40 from illo
// ...
});
let yTri = new Zdog.Polygon({
addTo: illo,
translate: { y: -60 }, // y -60 from illo
// ...
});
Copying
- You don’t need to create the same shape, you just simply .copy()


// create original
let rect = new Zdog.Rect({
addTo: illo,
width: 64,
height: 64,
translate: { x: -48 },
stroke: 16,
color: '#EA0',
});
// copy
rect.copy({
// overwrite original options
translate: { x: 48 },
color: '#C25',
});
copy with their children
// create original
let rect = new Zdog.Rect({
// ...
});
// add child item
new Zdog.Shape({
addTo: rect,
// ...
});
// copy rect and its children
rect.copyGraph({
// overwrite original rect options
translate: { x: 48 },
color: '#C25',
});
Groups
You can group nodes using .Group({})
// render shapes in order added
var eyeGroup = new Zdog.Group({
addTo: illo,
translate: { z: 20 },
});
// eye white first
new Zdog.Ellipse({
addTo: eyeGroup,
width: 160,
height: 80,
// ...
});
// then iris
let iris = new Zdog.Ellipse({
addTo: eyeGroup,
diameter: 70,
// ...
});
// then pupil
iris.copy({
diameter: 30,
color: '#636',
});
// highlight last in front
iris.copy({
diameter: 30,
translate: { x: 15, y: -15 },
color: 'white',
});
Many more in the documentation
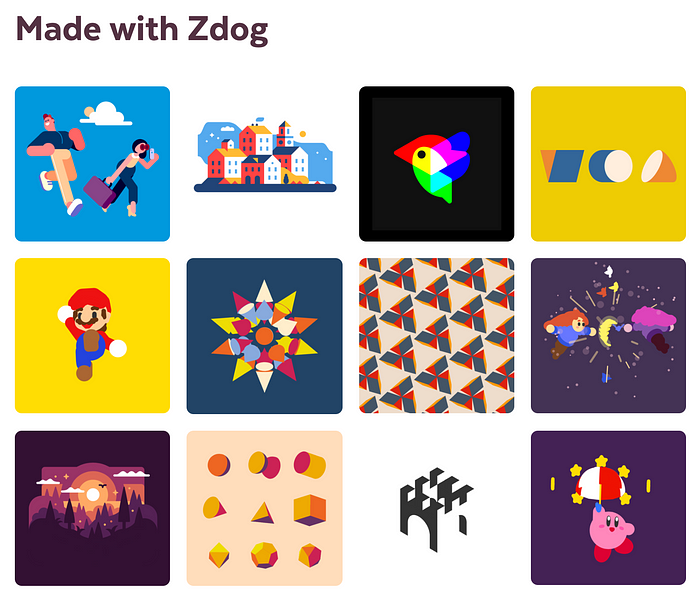
You can see many cool demos in the official website
Reference